iNTRO
Here we will see extra samples about dynamic C# capablities in terms of making code cleaner and simpler
Reflection Sample
Sometimes we need to use .NET Reflection for getting information about types dynamically (or interfaces, members, etc… ) contained into given assembly. Although .NET Reflection is a power technique for inspecting types, members, etc.. at Runtime there some reflection operations that are not recommended, like directly invokes over MethodInfo types…. The main reason for that is the performance. You can use a more efficient approach if you build a ExpressionTree taking the same MethodInfo as base, generate a lambda expression with it by calling to .Compile() method before running it. This will be considerably faster. Nevertheless, here we are going to provide a simple .NET Reflection sample for seeing how we can reduce it and make it cleaner by using dynamic
Let´s take a look into following .NET Reflection dummy code for invoking the CreatePerson() method over PersonHelper component:
This static method uses GetPerson() one, we can use something similar to
public static Person GetPerson()
{
return new Person
{
Name = "David",
Surname = "Checa",
Married = true,
Gender = Gender.Male,
DateOfBirth = new DateTime(1977, 5, 24),
Email = "david.checa@xyz.com",
City = new City()
{
Description = "Hortaleza (Madrid)",
Name = "Madrid",
},
Mobile = "000000000",
NIF = "AAAAAAAAAK",
Phone = "000000000",
PostalCode = "28341",
Country = new Country()
{
Name = "Spain",
}
};
}
At this point, we can use dynamic for rewriting code of Main() method in the following way
static void Main(string[] args)
{
Person person = GetPerson();
PersonHelper helper = new PersonHelper();
((dynamic)helper).CreatePerson(person);
}
Parsing JSON Sample
Finally we are going to see how can use dynamic for simplifying Json parsing scenarios. Let´s suppose that we have configured NewtonJson. Soft nugget package for making easier deserialization process
In this case, we assume that we have a json.txt file that contains a Person entity in json format, something like

If we apply pretty format we have
Dynamic C# Samples 5That will correspond to the strongly typed Person entity introduced previously, that has the following implementation:
public enum Gender
{
Male = 0,
Female = 1
}
public class Person
{
public Guid Id { get; set; }
public string Name { get; set; }
public string Surname { get; set; }
public Gender Gender { get; set; }
public bool Married { get; set; }
public DateTime DateOfBirth { get; set; }
public string NIF { get; set; }
public string Phone { get; set; }
public string Mobile{ get; set; }
public string FlattedAddress{ get; set; }
public string Email { get; set; }
public string PostalCode { get; set; }
public City City { get; set; }
public Country Country{ get; set; }
[DataType(DataType.MultilineText)]
public string Details { get; set; }
public byte[] Image { get; set; }
public string ImagePath { get; set; }
}
So from here we provide standard deserializing code Main() console method in the following way,
static void Main10(string[] args)
{
string json = string.Empty;
using (StreamReader r = new StreamReader(@"c:\activities\json.txt"))
json = r.ReadToEnd();
Person person = JsonConvert.DeserializeObject<Person>(json);
var details = person.Details;
var name = person.Name;
var surname = person.Surname;
var nif = person.NIF;
var phone = person.Phone;
var married = person.Married;
var postalCode = person.PostalCode;
// etc.....
Console.WriteLine(string.Format("person data : {0}name=[{1}],{0}surname=[{2}],{0}nif=[{3}], {0}phone=[{4}],{0}details=[{5}],{0}postalcode=[{6}]",
Environment.NewLine, name, surname, nif, phone,details, postalCode));
Console.ReadLine();
}
We can run the console obtaining
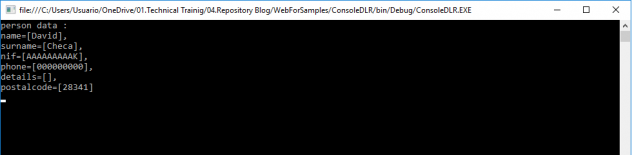
Well, at this point we can use dynamic C# for providing an alternative implementation for Main() where the strongly typed Person is not needed at all:
static void Main(string[] args)
{
string json = string.Empty;
using (StreamReader r = new StreamReader(@"c:\activities\json.txt"))
json = r.ReadToEnd();
dynamic person = JsonConvert.DeserializeObject(json);
var details = person.Details;
var name = person.Name;
var surname = person.Surname;
var nif = person.NIF;
var phone = person.Phone;
var married = person.Married;
var postalCode = person.PostalCode;
// etc.....
Console.WriteLine(string.Format("person data FROM DYNAMIC: {0}name=[{1}],{0}surname=[{2}],{0}nif=[{3}], {0}phone=[{4}],{0}details=[{5}],{0}postalcode=[{6}]",
Environment.NewLine, name, surname, nif, phone, details, postalCode));
Console.ReadLine();
}
Now, we run the console again, resulting:

So that´s all by now
See you on the road!